Chasing the Collatz Conjecture with Python: Adventures in Mathematics
- Anthony Mudhoka
- Apr 30, 2023
- 4 min read
Updated: May 5, 2023
One thing that has eluded me in the world of mathematics is the notorious Collatz conjecture. This simple pattern has led mathematicians astray since 1937. No one has been able to solve it, almost a century later. It even stumped the brilliant mathematician Paul Erdos. In case you are not familiar with the Collatz Conjecture, I already wrote about it in a previous blog, The Collatz Conjecture: The million dollars puzzle.
In this blog post I will make attempts to use python codes to uncover some of the patterns of the Collatz Conjecture. The codes are nothing groundbreaking but just tools to help us in our exploration, like a microscope to a biologist or a telescope to an astronomer.
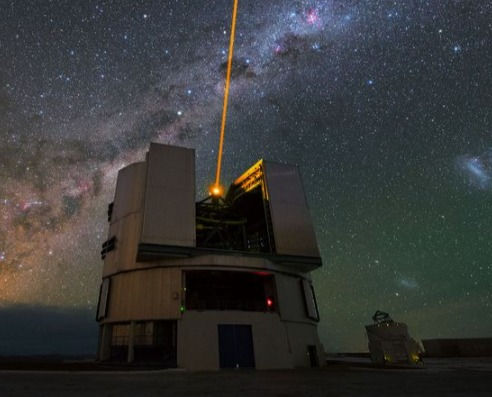
I have broken the codes into several chunks each performing a specific task. Feel free to try and even modify them yourself. If you have more ideas of your own that you need help to code, I can do it for you.
Just let me know on the contact form. I have coded several other modules related to the Collatz Conjecture.
Steps Counter
Starting out with the most basic one, this function will basically calculate the number of steps in a particular Collatz orbit with a given starting number.
def collatz(n):
steps = 0
while n != 1:
if n % 2 == 0:
n = n // 2
else:
n = 3 * n + 1
steps += 1
return steps
n = int(input("Enter a positive integer: "))
steps = collatz(n)
print("It took", steps, "steps to reach 1.")
2. Highest point in the orbit
This function searches for the maximum number reached in an orbit. Do not be surprised if the highest number is the same as the starting number. This scenario happens occasionally especially with large starting numbers and also when the starting number is a power of 2.
def collatz_sequence(n):
sequence = [n]
while n != 1:
if n % 2 == 0:
n = n // 2
else:
n = 3 * n + 1
sequence.append(n)
return sequence
start_num = int(input("Enter a positive integer to start the sequence: "))
sequence = collatz_sequence(start_num)
max_num = max(sequence)
print("The highest number in the sequence is:", max_num)
3. Collatz Sequence
This one lists all the steps in the Collatz sequence for a given starting number.
def collatz_sequence(n):
sequence = [n]
while n != 1:
if n % 2 == 0:
n = n // 2
else:
n = 3 * n + 1
sequence.append(n)
return sequence
start_num = int(input("Enter a positive integer to start the sequence: "))
sequence = collatz_sequence(start_num)
print("The Collatz sequence for", start_num, "is:")
for num in sequence:
print(num)
4. Sequence Grapher
Would it not be fun to visualize the erratic behavior of the Collatz Conjecture on a graph? This function does exactly what the previous one does, except that this one graphs each step on a graph. Make sure you have the matplotlib module installed on your Python IDE. You can check my previous blog on python modules, link here, for instructions on how to install python modules.
import matplotlib.pyplot as plt
def collatz_sequence(n):
sequence = [n]
while n != 1:
if n % 2 == 0:
n = n // 2
else:
n = 3 * n + 1
sequence.append(n)
return sequence
start_num = int(input("Enter a positive integer to start the sequence: "))
sequence = collatz_sequence(start_num)
plt.plot(sequence)
plt.title("Collatz Sequence for " + str(start_num))
plt.xlabel("Step")
plt.ylabel("Number")
plt.show()
5. Even-Odd Ratio
This one sorts out all the steps and calculates the number of odds and evens that appeared in a particular sequence. It shouldn't be a surprise that all the powers of 2 will have only have one odd, which is 1 at the end.
def collatz_sequence(n):
sequence = [n]
while n != 1:
if n % 2 == 0:
n = n // 2
else:
n = 3 * n + 1
sequence.append(n)
return sequence
start_num = int(input("Enter a positive integer to start the sequence: "))
sequence = collatz_sequence(start_num)
evens = len([num for num in sequence if num % 2 == 0])
odds = len(sequence) - evens
print("The Collatz sequence for", start_num, "contains", evens, "even numbers and", odds, "odd numbers.")
6. Longest Orbit up to a limit
This one computes all all the sequences of starting numbers up to a specified limit then finds the starting number with the most number of steps. Warning : This computation can take relatively more computation power since it is highly iterative in nature. Edit: I realized we could make the code below work more efficiently by taking out some of the redundant computations. We could create a set that holds all the steps in an orbit then stop the computation once an overlap with previous orbits has been established.
def collatz_length(n):
length = 0
while n != 1:
if n % 2 == 0:
n //= 2
else:
n = 3*n + 1
length += 1
return length
limit = int(input("Enter a limit: "))
longest_sequence = 0
start_num = 0
for i in range(1, limit+1):
sequence_length = collatz_length(i)
if sequence_length > longest_sequence:
longest_sequence = sequence_length
start_num = i
print("The starting number that generates the longest Collatz sequence up to", limit, "is", start_num, "with a length of", longest_sequence)
"Exploration is really the essence of the human spirit. And to pause, to falter, to turn our back on the quest for knowledge, is to perish." - Frank Borman
Comentarios